Intro to Physical Computing
Jeff Feddersen
Spring 2003
A very common problem faced by programmers is that of remapping
an input in one range to an output in another range:
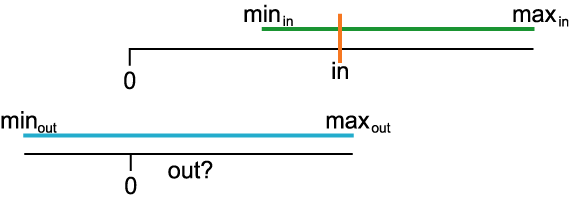
This is the code to remap the input to the output:
on shortRemap inputValue, min1, max1,
min2, max2
return
(float(inputValue) - min1)/(max1 - min1) * (max2 - min2) + min2
end
And here's an explanation of how it works:
on remap inputValue, min1, max1, min2,
max2
--
map a value between min1 and max1 to min2 and max2
--
Step 1: calculate the total range between the two mins and maxes
totalInputRange =
max1 - min1
totalOutputRange =
max2 - min2
--
Step 2: "normalize" input value by sliding it to 0
normalizedInputValue
= inputValue - min1
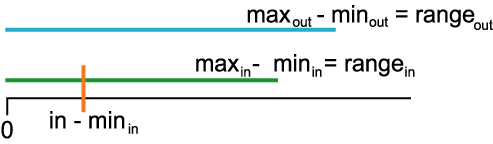
--
Step 3: calculate input value as a percentage of the total input range
percentageValue =
float(normalizedInputValue) / float(totalInputRange)
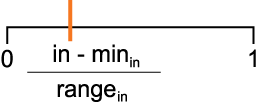
--
Step 4: apply that percentage to the output range
normalizedOutputValue
= percentageValue * totalOutputRange
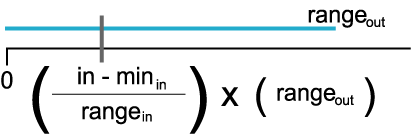
--
Step 4: "unnormalize" the output value by sliding it from
0 to the output minimum
outputValue = normalizedOutputValue
+ min2
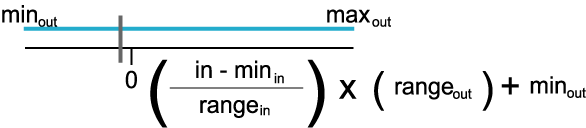
return
outputValue
end
|